Build and scale Web3 games, with ease
Everything you need for Web3 gaming growth and monetization. Multichain and real cross-console support with embedded user onboarding.

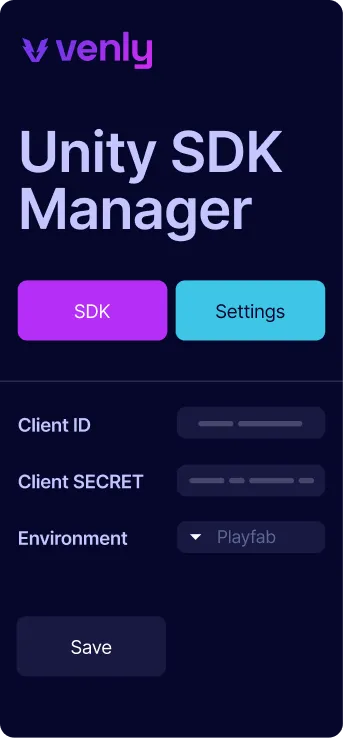

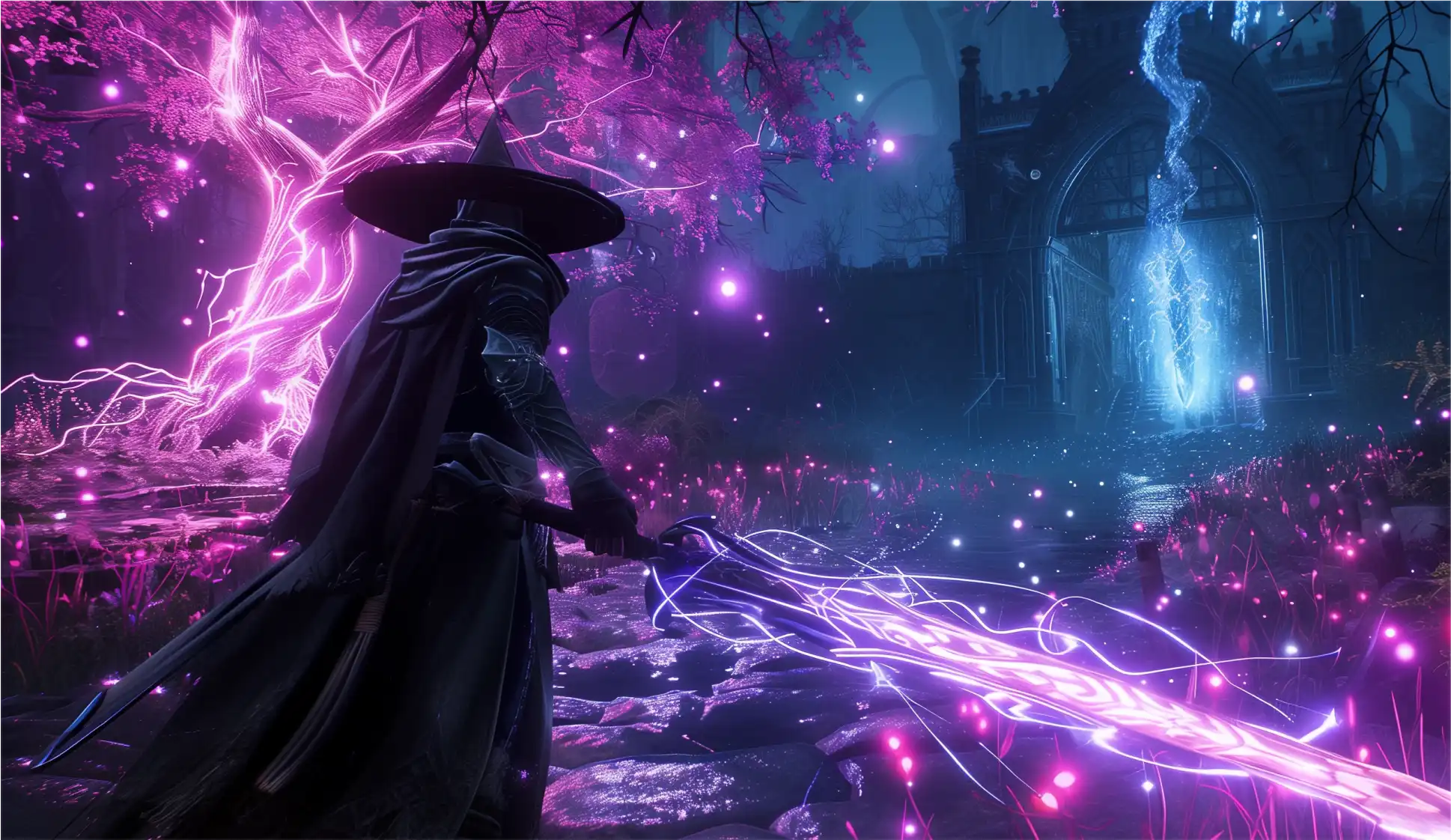

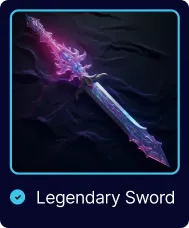
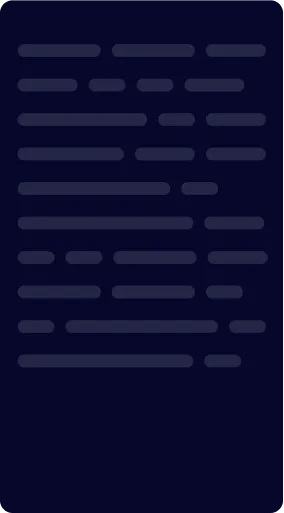
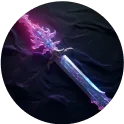

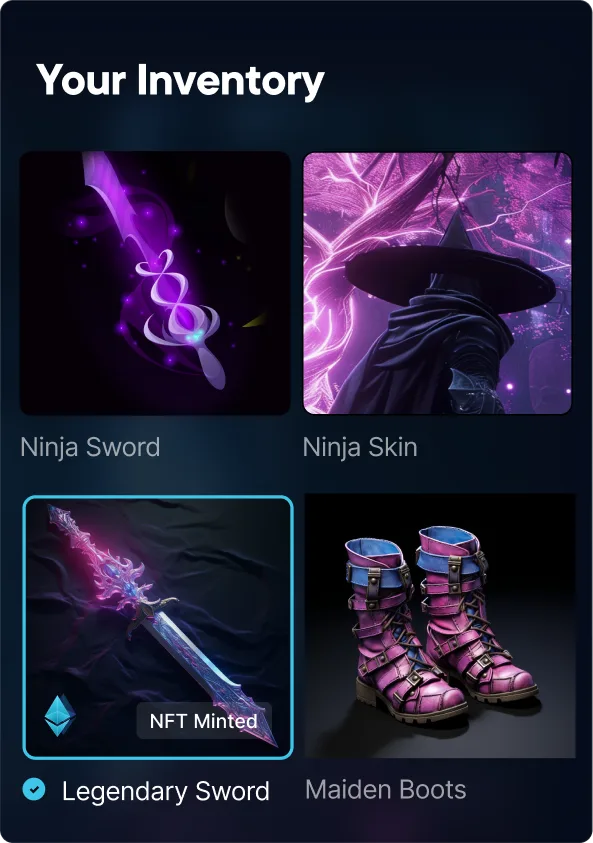



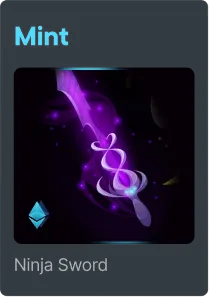
Build, scale & engage with Venly
Access all gaming API functionalities directly within Unity and Unreal, the industry's leading game development engines. Our toolkit is a natural extension of your development environment.

Integrate with Beamable, Playfab, or your custom backend to add LiveOps services to your project. Everything you need to operate and monetize your game.

With external smart contract support, you have the freedom to implement complex game mechanics and tokenomics. Our toolkit is compatible with popular token types like ERC20, ERC1155, and ERC72.
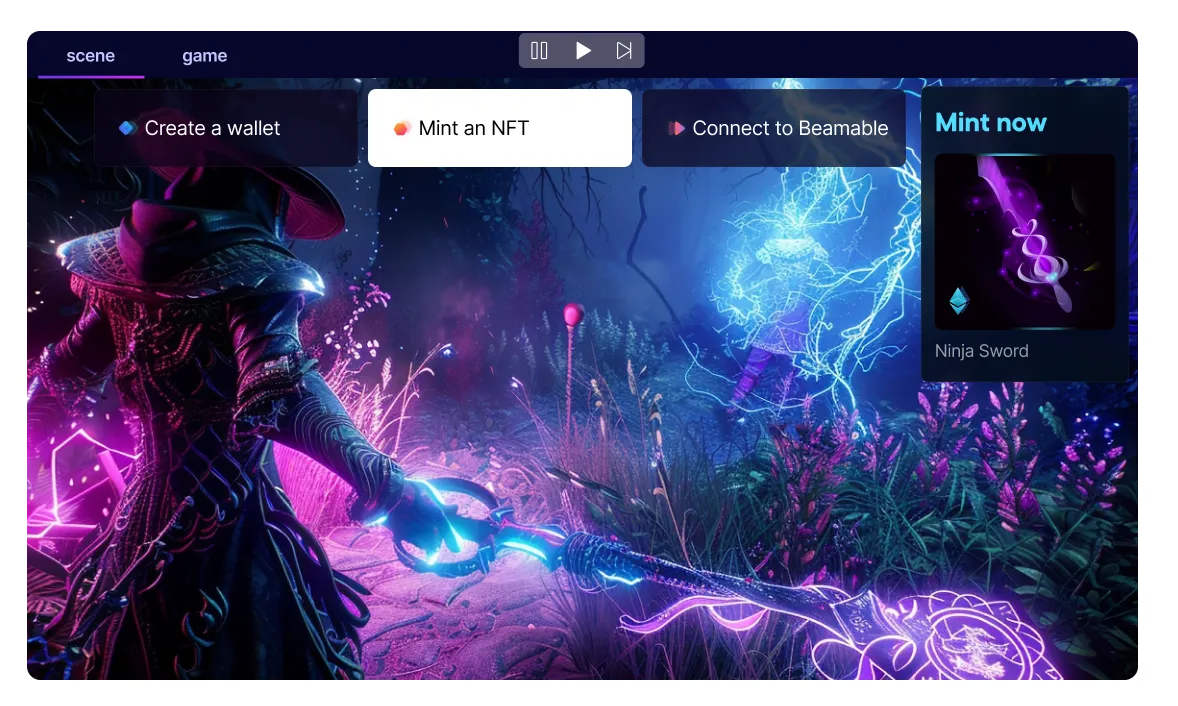
Expand your reach across platforms


//**Request Parameters
VyCreateWalletRequest request = new()
{
Chain = eVyChain.Matic,
Description = "My first wallet",
Pincode = "123456",
WalletType = eVyWalletType.ApiWallet
};
//**Execute Request
//Option 1 (Promise-like)
VenlyAPI.Wallet.CreateWallet(request)
.OnSuccess(wallet =>
{
//Wallet Created!
})
.OnFail(exception =>
{
//Oops, something went wrong...
});
//Option 2 (async/await)
var wallet = await VenlyAPI.Wallet.CreateWallet(request).AwaitResult();
//**Header
#include "AutoGen\VyWalletAPI.h"
//**Request Parameters
FVyCreateWalletRequest Params{};
Params.Chain = EVyChain::MATIC;
Params.Description = FVyOptString(TEXT("My First Wallet"));
Params.Pincode = TEXT("123456");
//**OnComplete Handler
FVyOnCreateWalletComplete OnComplete;
OnComplete.BindLambda([](FVyCreateWalletResponse Response)
{
if(Response.Success) {/*Wallet Created!*/}
else {/*Oops, something went wrong...*/}
});
//**Execute Request
UVyWalletAPI::Get()->CreateWallet(Params, OnComplete);
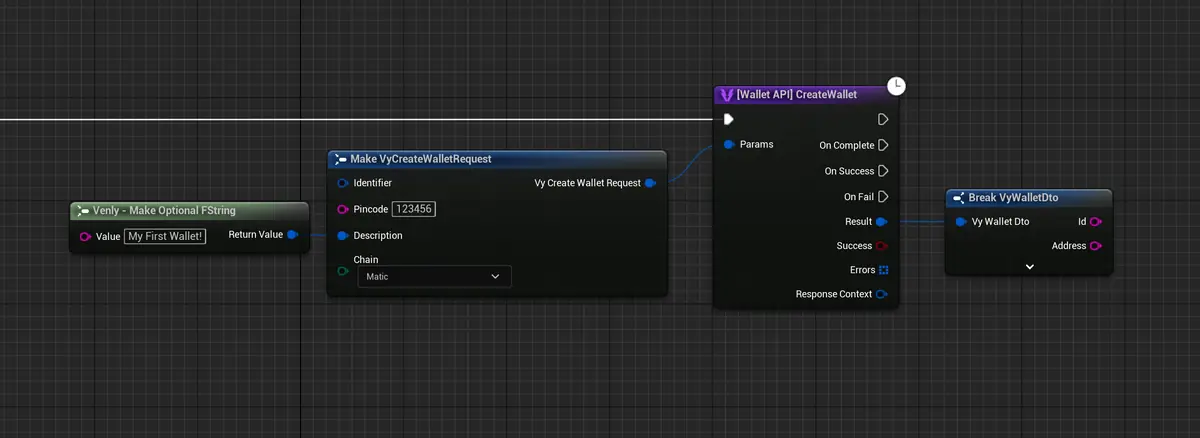
//**Request Parameters (0.5 Matic to target address)
VyTransactionNativeTokenTransferRequest request = new()
{
Chain = eVyChain.Matic,
WalletId = "1f2924a2-22a8-47b8-a93f-80d7217ee6b0",
ToAddress = "0xec1955c7E899e7aef312B48e4559E5A321504253",
Value = 0.5
};
//**Execute Request (Pin Required)
//Option 1 (Promise-like)
VenlyAPI.Wallet.ExecuteNativeTokenTransfer(pincode:"123456", request)
.OnSuccess(txResult =>
{
//Transfer Executed!
})
.OnFail(exception =>
{
//Oops, something went wrong...
});
//Option 2 (async/await)
var txResult = await VenlyAPI.Wallet.ExecuteNativeTokenTransfer("123456", request).AwaitResult();
//**Header
#include "AutoGen\VyWalletAPI.h"
//**Request Parameters
FVyTransferNativeTokenRequest Params{};
Params.Pincode = TEXT("123456");
Params.TransactionRequest.Chain = EVyChain::MATIC;
Params.TransactionRequest.WalletId = TEXT("1f2924a2-22a8-47b8-a93f-80d7217ee6b0");
Params.TransactionRequest.ToAddress = TEXT("0xec1955c7E899e7aef312B48e4559E5A321504253");
Params.TransactionRequest.Value = FVyOptDouble(0.5);
//**OnComplete Handler
FVyOnTransferNativeTokenComplete OnComplete;
OnComplete.BindLambda([](FVyTransferNativeTokenResponse Response)
{
if(Response.Success) {/*Transfer Executed!*/}
else {/*Oops, something went wrong...*/}
});
//**Execute Request
UVyWalletAPI::Get()->TransferNativeToken(Params, OnComplete);
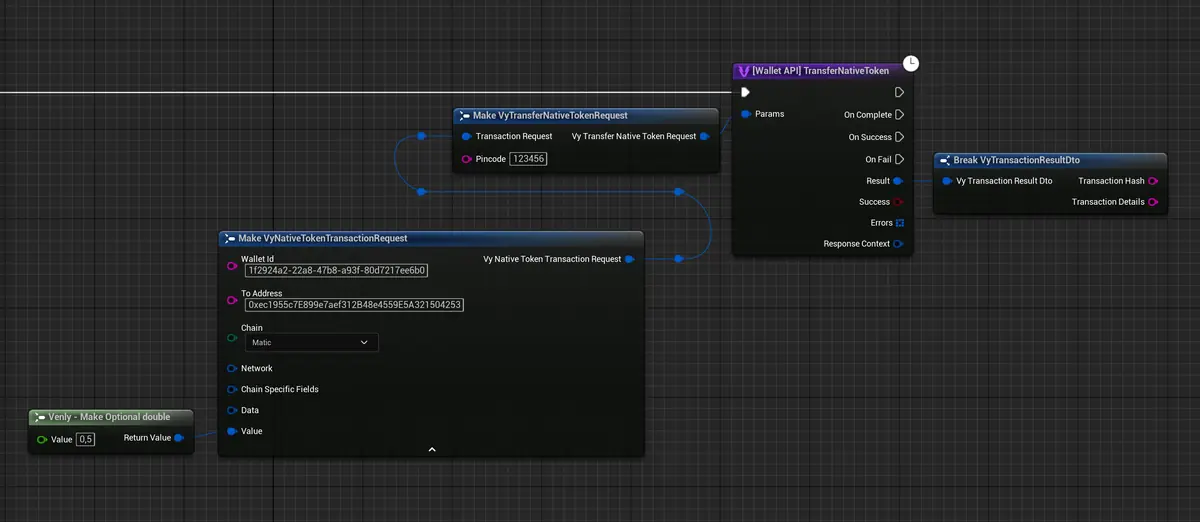
//**Request Parameters (Destinations & Amounts)
VyMintTokensRequest request = new()
{
Destinations = new VyTokenDestinationDto[]
{
new VyTokenDestinationDto
{
Address = "0xec1955c7E899e7aef312B48e4559E5A321504253",
Amount = 1
}
}
};
//**Execute Request (ContractId & TokenTypeId Required)
//Option 1 (Promise-like)
VenlyAPI.Nft.MintTokens(contractId:52415, typeId:5, request)
.OnSuccess(mintedTokens =>
{
//Mint Initiated!
})
.OnFail(exception =>
{
//Oops, something went wrong...
});
//Option 2 (async/await)
var mintedTokens = await VenlyAPI.Nft.MintTokens(52415, 5, request).AwaitResult();
//**Header
#include "AutoGen\VyNftAPI.h"
//**Request Parameters
FVyMintTokensRequest Params{};
Params.Destinations.Add(FVyTokenDestinationDto{});
Params.Destinations[0].Address = TEXT("0xec1955c7E899e7aef312B48e4559E5A321504253");
Params.Destinations[0].Amount = 1;
//**OnComplete Handler
FVyOnMintTokensComplete OnComplete;
OnComplete.BindLambda([](FVyMintTokensResponse Response)
{
if(Response.Success) {/*Mint Initiated!*/}
else {/*Oops, something went wrong...*/}
});
//**Execute Request
UVyNftAPI::Get()->MintTokens(52415, 5, Params, OnComplete);
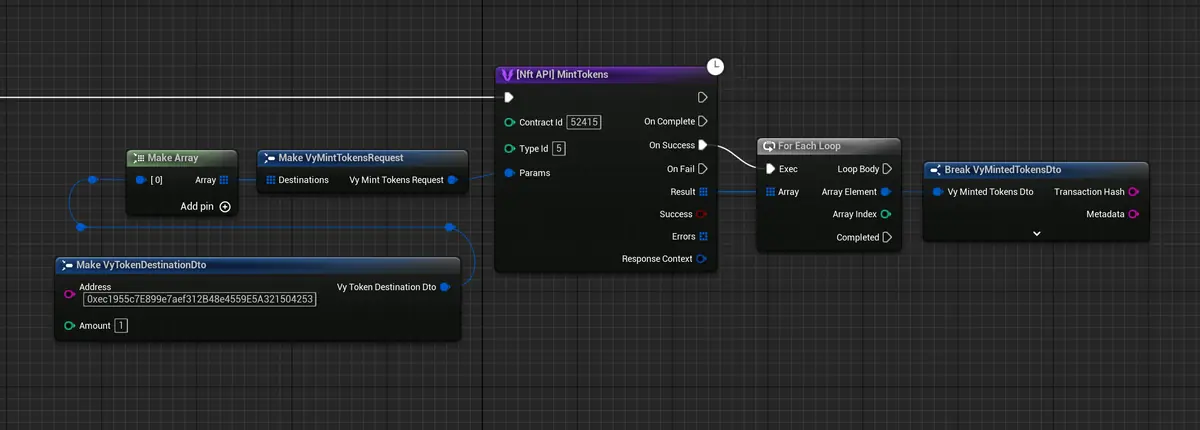
//**Request Parameters (Define Sale/Auction Offer)
//Ext > Handles entire flow (Creation/Signing/Custody)
//Offers from external wallets are also possible
VySaleOfferRequestExt request = new()
{
Pincode = "123456",
WalletId = "1f2924a2-22a8-47b8-a93f-80d7217ee6b0",
OfferRequest = new VySaleOfferRequest
{
SellerAddress = "0xec1955c7E899e7aef312B48e4559E5A321504253",
Visibility = eVyVisibilityType.Public,
Price = 0.5,
Nft = new VyNftDto
{
Chain = eVyChain.Matic,
Address = "0xdbd6fef0a23f64a2b17749bba4232256475dcfb9",
TokenId = "2"
}
//extra options...
}
};
//**Execute Request
//Option 1 (Promise-like)
VenlyAPI.Market.CreateOfferExt(request)
.OnSuccess(saleOffer =>
{
//Sale Offer Created!
})
.OnFail(exception =>
{
//Oops, something went wrong...
});
//Option 2 (async/await)
var saleOffer = await VenlyAPI.Market.CreateOfferExt(request).AwaitResult();
//**Header
#include "AutoGen\VyMarketAPI.h"
//**Request Parameters
FVySaleOfferRequest Params{};
Params.SellerAddress = TEXT("0xec1955c7E899e7aef312B48e4559E5A321504253");
Params.Visibility = FVyOptVisibilityType(EVyVisibilityType::PUBLIC);
Params.Price = FVyOptDouble(0.5);
Params.Nft.Chain = EVyChain::MATIC;
Params.Nft.Address = TEXT("0xdbd6fef0a23f64a2b17749bba4232256475dcfb9");
Params.Nft.TokenId = TEXT("2");
//extra options...
//**OnComplete Handler
FVyOnCreateSaleOfferComplete OnComplete{};
OnComplete.BindLambda([](FVyCreateSaleOfferResponse Response)
{
if(Response.Success) {/*Sale Offer Created!*/}
else {/*Oops, something went wrong...*/}
});
//**Execute Request
UVyMarketAPI::Get()->CreateSaleOffer(Params, OnComplete);
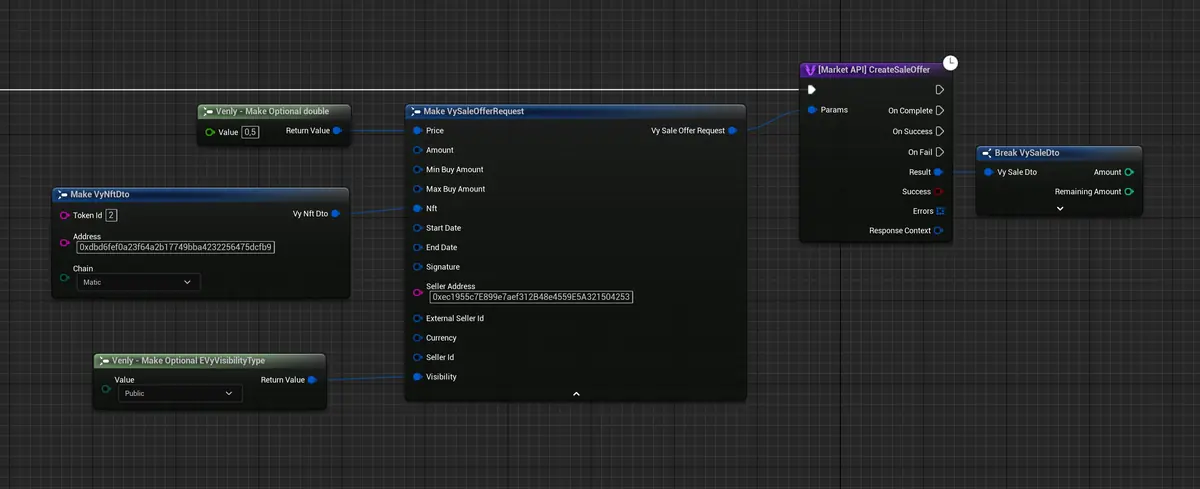
//**Retrieve a wallet linked to a logged-in user
//managed by an external Identity Service (Beamable/PlayFab/Custom)
//Option 1 (Promise-like)
VenlyAPI.ProviderExtensions.GetWalletForUser()
.OnSuccess(wallet =>
{
//Wallet Retrieved!
})
.OnFail(exception =>
{
//Oops, something went wrong...
});
//Option 2 (async/await)
var wallet = await VenlyAPI.ProviderExtensions.GetWalletForUser();
//**Header
#include "VyProviderExtensions.h"
//**Retrieve a wallet linked to a logged-in user
//managed by an external Identity Service (Beamable/PlayFab)
//**OnComplete Handler
FVyOnGetWalletForUserComplete OnComplete{};
OnComplete.BindLambda([](FVyGetWalletResponse Response)
{
if(Response.Success) {/*Wallet Retrieved!*/}
else {/*Oops, something went wrong...*/}
});
//**Execute Request
UVyProviderExtensions::Get()->CPP_GetWalletForUser(OnComplete);
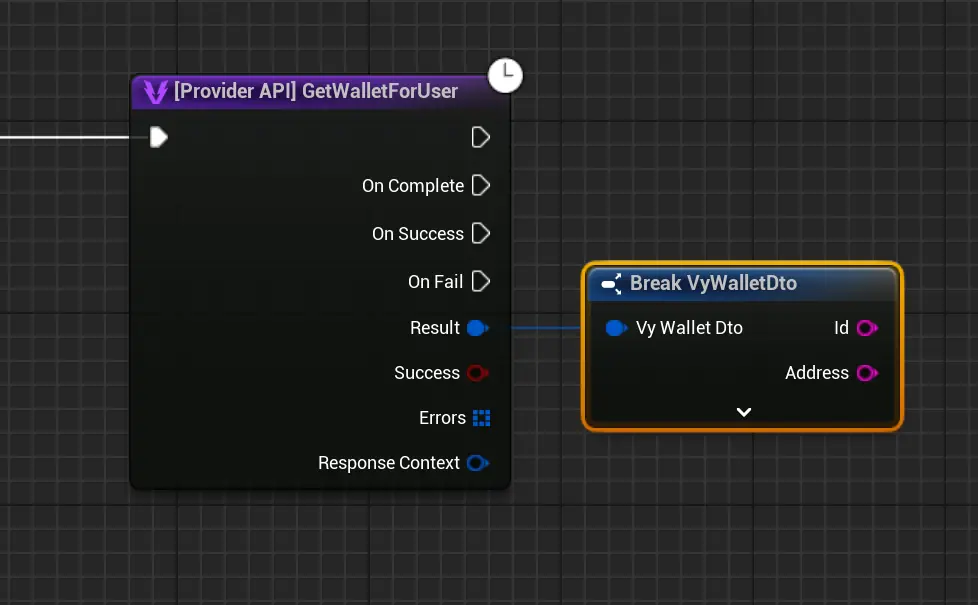
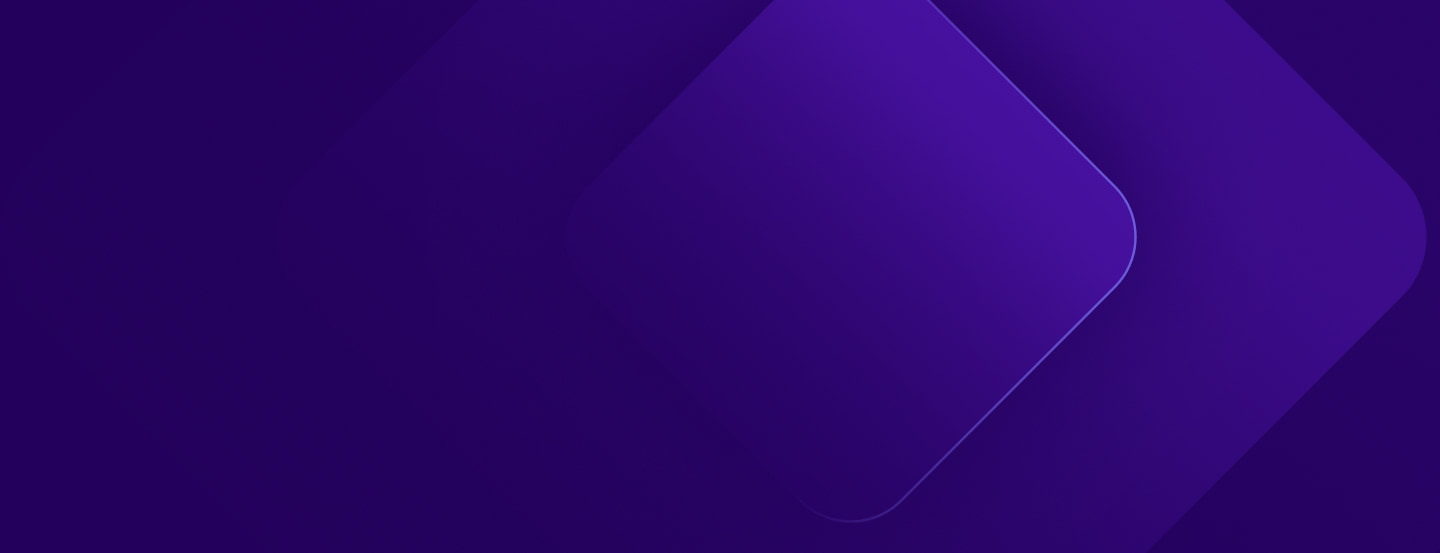